
I’d like to suggest a small change to how class constructors work, particularly for data classes. To make code like this correct for null values, use the nullable type on the right-hand side of the cast: val x: String y as String 'Safe' (nullable) cast operator To avoid exceptions, use the safe cast operator as, which returns null on failure. Null Safety In Java, all objects were allowed to be nullable. I am in the process of converting a large Java code base to Kotlin, and I’ve run into an issue that makes this transition less than smooth. I consider Kotlin to be a very superior language, and it is also my favourite. As we can see in the code above, let2() is a generic function, so the two parameters’ (p1 and p2) types can be different. block is a function that accepts two not-null arguments and returns a nullable R instance. p1 and p2 are the two nullable parameters. Otherwise, structural equality is used, which disagrees with the standard so that NaN is equal to itself, NaN is considered greater than any other element, including POSITIVE_INFINITY, and -0.0 is not equal to 0.0.Hi there. The function let2() has three parameters: p1, p2, and block. When an equality check operands are statically known to be Float or Double (nullable or not), the check follows the IEEE 754 Standard for Floating-Point Arithmetic. Kotlin’s type system distinguishes between nullable and non-nullable types, enabling built-in null safety checks and providing concise syntax for handling nullable values. In this article, we’ve taken a look at different ways we can default a null value for a non-optional parameter. For values represented by primitive types at runtime (for example, Int), the = equality check is equivalent to the = check. a = b evaluates to true if and only if a and b point to the same object. Referential equality is checked by the = operation and its negated counterpart !=. Structural equality has nothing to do with comparison defined by the Comparable interface, so only a custom equals(Any?) implementation may affect the behavior of the operator.
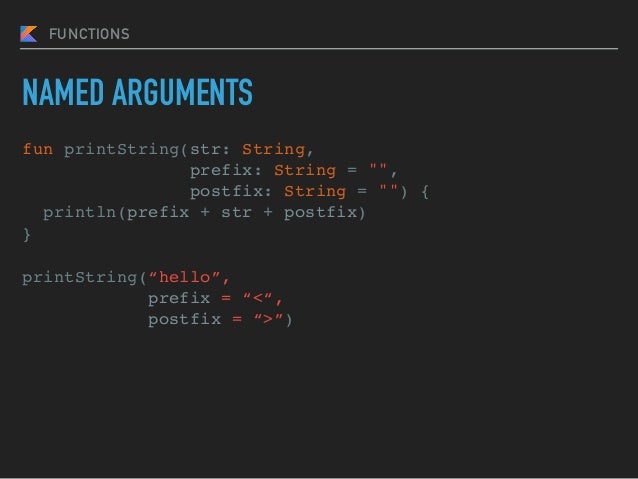
Functions with the same name and other signatures, like equals(other: Foo), don't affect equality checks with the operators = and !=. To provide a custom equals check implementation, override the equals(other: Any?): Boolean function.
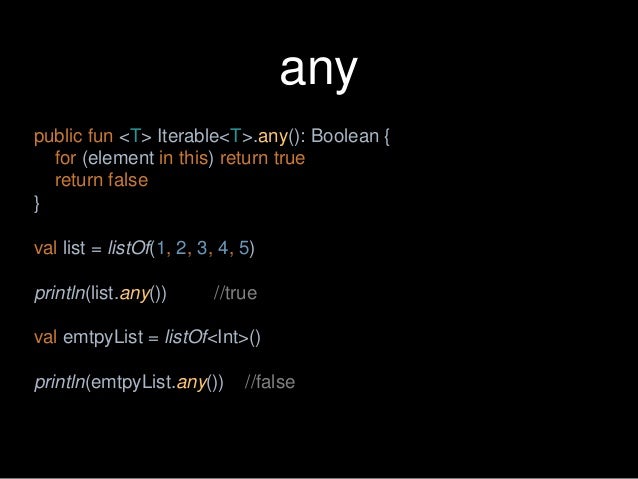
Note that there's no point in optimizing your code when comparing to null explicitly: a = null will be automatically translated to a = null.
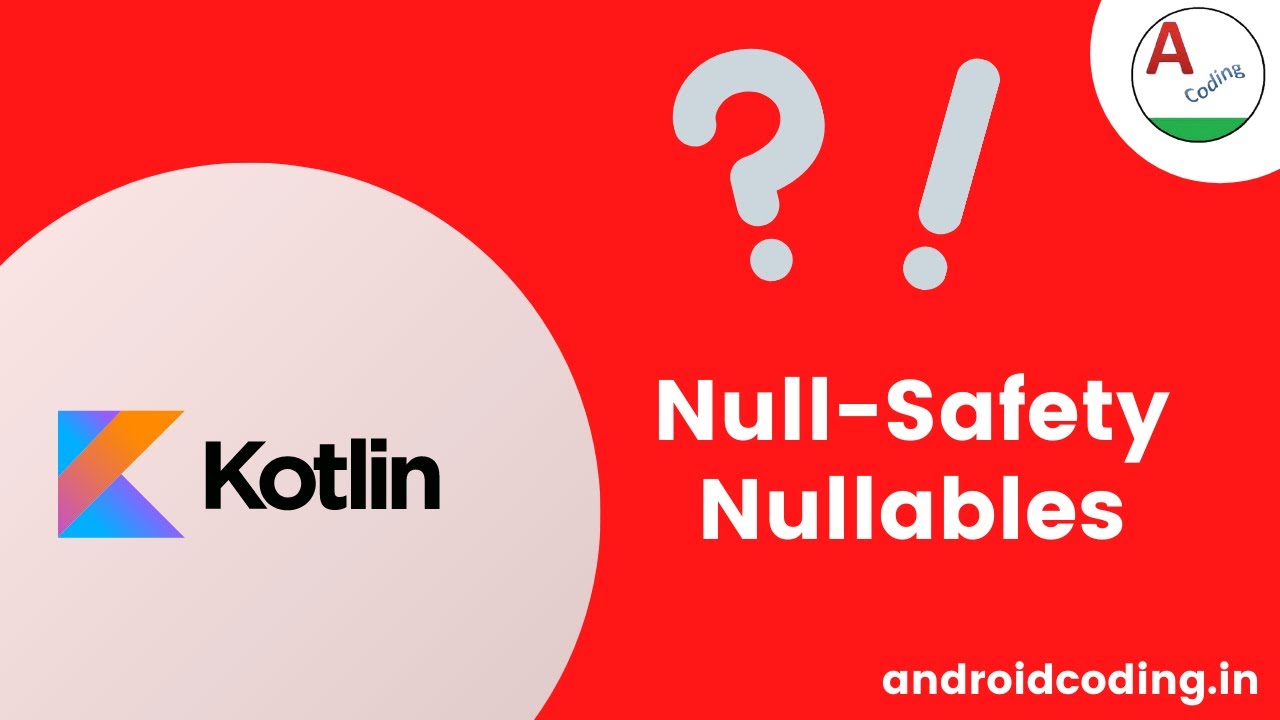
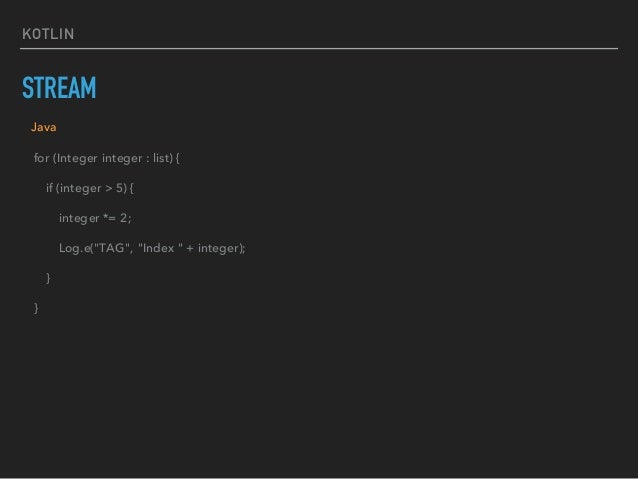
If a is not null, it calls the equals(Any?) function, otherwise ( a is null) it checks that b is referentially equal to null.
